React from the background of an Angular developer
So, I’ve been playing around with Angular for more than 7 years now, and I’ve always wanted to dig deep into React but my mentality always takes me to Angular. But finally, I’ve been able to know some stuff and here’s what I learnt so far.
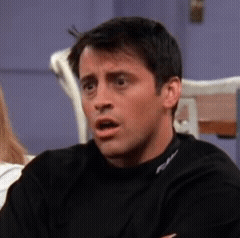
Inputs in Angular and React
So, inputs in Angular are props
in React, so as an example:
Angular:
// Angular Component
import { Component, Input } from '@angular/core';
@Component({
selector: 'app-child',
template: '<p>{{ inputData }}</p>',
})
export class ChildComponent {
@Input() inputData: string;
}
React:
// React Component
import React from 'react';
const ChildComponent = (props) => {
return <p>{props.inputData}</p>;
};
export default ChildComponent;
// Parent Component in React
import React from 'react';
import ChildComponent from './ChildComponent';
const ParentComponent = () => {
const dataToPass = 'Hello from parent!';
return <ChildComponent inputData={dataToPass} />;
};
export default ParentComponent;
Calling a Service
In Angular, it is common to utilize services for the purpose of retrieving and overseeing data. These services can be perceived as a means to encapsulate the intricacies of business logic and data manipulation. Once the data has been obtained through a service, it is then possible to establish a connection between said data and the visual representation by means of properties within the component.
Here’s a simplified example in Angular:
// Angular Service
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root',
})
export class DataService {
constructor(private http: HttpClient) {}
getData(): Observable<any> {
return this.http.get('https://api.example.com/data');
}
}
// Angular Component using the service
import { Component, OnInit } from '@angular/core';
import { DataService } from './data.service';
@Component({
selector: 'app-example',
template: '<p>{{ data }}</p>',
})
export class ExampleComponent implements OnInit {
data: any;
constructor(private dataService: DataService) {}
ngOnInit(): void {
this.dataService.getData().subscribe((result) => {
this.data = result;
});
}
}
In React, when working with data management and updates, you have the option to use either component state or state management libraries such as Redux or Context API. Let me provide you with a simplified example of how this can be done using component state in React:
// React Component using component state
import React, { useState, useEffect } from 'react';
const ExampleComponent = () => {
const [data, setData] = useState(null);
useEffect(() => {
const fetchData = async () => {
const response = await fetch('https://api.example.com/data');
const result = await response.json();
setData(result);
};
fetchData();
}, []);
return <p>{data}</p>;
};
export default ExampleComponent;
The fetched data is stored in the data state in this React example. When the component mounts, the useEffect hook fetches data.
Angular and React, with differing specific implementation details, both offer mechanisms that could be loosely compared to models in other frameworks, allowing for the management and manipulation of data.
Variables
Both Angular and React leÂt you store and manage data with variables inside components. But, their ways of using variables vary.
Angular:
When working with Angular, it’s common to declare variables within a component using class properties. Here’s a simple example for illustration:
// Angular Component
import { Component } from '@angular/core';
@Component({
selector: 'app-example',
template: '<p>{{ myVariable }}</p>',
})
export class ExampleComponent {
myVariable: string = 'Hello from Angular!';
}
In today’s React, state management is frequently handled through functional components and hooks. A comparable illustration of this would be implementing a functional component with the useState hook.
// React Functional Component with Hooks
import React, { useState } from 'react';
const ExampleComponent = () => {
const [myVariable, setMyVariable] = useState('Hello from React!');
return <p>{myVariable}</p>;
};
export default ExampleComponent;
To demonstrate, myVariable is a state variable and setMyVariable is the function used to modify it. These are important components of the React Hooks API. Overall, although both Angular and React provide the ability to utilize variables within components, they diverge in their approaches for declaring and handling them.
Conclusion
To be honest I loved React a little, still, my loyalty is to Angular 😀 but, I will definitely keep going deeper into it.
Meanwhile, check my other FrontEnd topics.
And, here’s a dancing potato
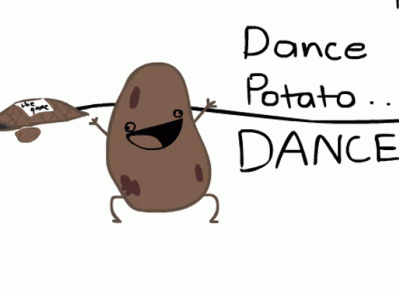