Angular using Vanilla JavaScript Libraries
Have you ever wanted a component in Angular and you’ve never found it on npm or github for any reason? You keep searching and searching, and you find it, but Oh ! it’s written in javascript, not angular π₯², What should we do now? You ever thought of how to add vanilla javascript libraries in angular?
How do we do so?
We have three ways to add vanilla javascript libraries in angular.
I will take Tabulator as an example to demonstrate and demo.
Angular index.html
As per the Tabulator documentation, you can just add the CSS and Javascript with the CDN like this
<link href="https://unpkg.com/tabulator-tables@5.5.0/dist/css/tabulator.min.css" rel="stylesheet">
<script type="text/javascript" src="https://unpkg.com/tabulator-tables@5.5.0/dist/js/tabulator.min.js"></script>
And then what? the documentation states that you should do something like this:
<div id="example-table"></div>
<script>
//define data array
var tabledata = [
{id:1, name:"Oli Bob", progress:12, gender:"male", rating:1, col:"red", dob:"19/02/1984", car:1},
{id:2, name:"Mary May", progress:1, gender:"female", rating:2, col:"blue", dob:"14/05/1982", car:true},
{id:3, name:"Christine Lobowski", progress:42, gender:"female", rating:0, col:"green", dob:"22/05/1982", car:"true"},
{id:4, name:"Brendon Philips", progress:100, gender:"male", rating:1, col:"orange", dob:"01/08/1980"},
{id:5, name:"Margret Marmajuke", progress:16, gender:"female", rating:5, col:"yellow", dob:"31/01/1999"},
{id:6, name:"Frank Harbours", progress:38, gender:"male", rating:4, col:"red", dob:"12/05/1966", car:1},
];
//initialize table
var table = new Tabulator("#example-table", {
data:tabledata, //assign data to table
autoColumns:true, //create columns from data field names
});
</script>
Ok, well that looks ok, but it’s not what we wanna do, we need to add it in our Angular component, right?
How? let’s assume we have a component for our table, We should do two things:
- Add our div in the component.html
- Add our javascript
In our index.html we will add our script and styles files, like so:
<link href="https://unpkg.com/tabulator-tables@5.5.0/dist/css/tabulator.min.css" rel="stylesheet">
<script type="text/javascript" src="https://unpkg.com/tabulator-tables@5.5.0/dist/js/tabulator.min.js"></script>
In our component.html, we will add our div, like so:
<div #table></div>
Notice the #table, so we will use ViewChild to reference our element as we cannot use selectors to reference our elements, because it can be duplicated in the page and things mess up
So, in out component.ts, we do the following:
import {
AfterViewInit,
Component,
ElementRef,
OnInit,
VERSION,
ViewChild,
} from '@angular/core';
declare const Tabulator: any;
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
})
export class AppComponent implements AfterViewInit {
@ViewChild('table') table: ElementRef;
ngAfterViewInit() {
//define data array
var tabledata = [
{
id: 1,
name: 'Oli Bob',
progress: 12,
gender: 'male',
rating: 1,
col: 'red',
dob: '19/02/1984',
car: 1,
},
{
id: 2,
name: 'Mary May',
progress: 1,
gender: 'female',
rating: 2,
col: 'blue',
dob: '14/05/1982',
car: true,
},
{
id: 3,
name: 'Christine Lobowski',
progress: 42,
gender: 'female',
rating: 0,
col: 'green',
dob: '22/05/1982',
car: 'true',
},
{
id: 4,
name: 'Brendon Philips',
progress: 100,
gender: 'male',
rating: 1,
col: 'orange',
dob: '01/08/1980',
},
{
id: 5,
name: 'Margret Marmajuke',
progress: 16,
gender: 'female',
rating: 5,
col: 'yellow',
dob: '31/01/1999',
},
{
id: 6,
name: 'Frank Harbours',
progress: 38,
gender: 'male',
rating: 4,
col: 'red',
dob: '12/05/1966',
car: 1,
},
];
//initialize table
var table = new Tabulator(this.table.nativeElement, {
data: tabledata, //assign data to table
autoColumns: true, //create columns from data field names
});
}
}
Notice few things:
- We used ElementRef instead the id to reference our div element
- we used declare const Tabulator, to tell Angular2+ that we have a class called Tabulator that you know nothing about, but … believe me, we have something called Tabulator ! πππ, without it, Angular would give us an error that it doesn’t know anything about Tabulator
angular.json
It’s pretty much the same as the index.html one, but instead of importing our styles and scripts in the index.html file, we will import them in our angular.json file and will also download them using npm
so we will just change our angular.json to be
{
"version": 1,
"newProjectRoot": "projects",
"projects": {
"codesandbox": {
"root": "",
"sourceRoot": "src",
"projectType": "application",
"prefix": "app",
"schematics": {},
"architect": {
"build": {
"builder": "@angular-devkit/build-angular:browser",
"options": {
"outputPath": "dist/codesandbox",
"index": "src/index.html",
"main": "src/main.ts",
"polyfills": "src/polyfills.ts",
"tsConfig": "src/tsconfig.app.json",
"assets": ["src/favicon.png", "src/assets"],
"styles": [
"node_modules/tabulator-tables/dist/css/tabulator.min.css",
"src/styles.css"
],
"scripts": [
"node_modules/tabulator-tables/dist/js/tabulator.min.js"
]
},
"configurations": {
"production": {
"fileReplacements": [
{
"replace": "src/environments/environment.ts",
"with": "src/environments/environment.prod.ts"
}
],
"optimization": true,
"outputHashing": "all",
"sourceMap": false,
"extractCss": true,
"namedChunks": false,
"aot": true,
"extractLicenses": true,
"vendorChunk": false,
"buildOptimizer": true
}
}
},
"serve": {
"builder": "@angular-devkit/build-angular:dev-server",
"options": {
"browserTarget": "codesandbox:build"
},
"configurations": {
"production": {
"browserTarget": "codesandbox:build:production"
}
}
},
"extract-i18n": {
"builder": "@angular-devkit/build-angular:extract-i18n",
"options": {
"browserTarget": "codesandbox:build"
}
},
"test": {
"builder": "@angular-devkit/build-angular:karma",
"options": {
"main": "src/test.ts",
"polyfills": "src/polyfills.ts",
"tsConfig": "src/tsconfig.spec.json",
"karmaConfig": "src/karma.conf.js",
"styles": ["src/styles.css"],
"scripts": [],
"assets": ["src/favicon.png", "src/assets"]
}
},
"lint": {
"builder": "@angular-devkit/build-angular:tslint",
"options": {
"tsConfig": [
"src/tsconfig.app.json",
"src/tsconfig.spec.json"
],
"exclude": ["**/node_modules/**"]
}
}
}
},
"codesandbox-e2e": {
"root": "e2e/",
"projectType": "application",
"architect": {
"e2e": {
"builder": "@angular-devkit/build-angular:protractor",
"options": {
"protractorConfig": "e2e/protractor.conf.js",
"devServerTarget": "codesandbox:serve"
},
"configurations": {
"production": {
"devServerTarget": "codesandbox:serve:production"
}
}
},
"lint": {
"builder": "@angular-devkit/build-angular:tslint",
"options": {
"tsConfig": "e2e/tsconfig.e2e.json",
"exclude": ["**/node_modules/**"]
}
}
}
}
},
"defaultProject": "codesandbox"
}
Import
Here we skip the angular,json and index.html files and we use npm with import
Let’s start by installing tabulator with
npm install tabulator-tables
and then in our component.ts file we do the following
— But !! we leave the css import in angular.json, don’t remove it
So, our component.ts is:
import {
AfterViewInit,
Component,
ElementRef,
OnInit,
VERSION,
ViewChild
} from '@angular/core';
import * as Tabulator from 'tabulator-tables';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements AfterViewInit {
@ViewChild('table') table: ElementRef;
ngAfterViewInit() {
//define data array
var tabledata = [
{
id: 1,
name: 'Oli Bob',
progress: 12,
gender: 'male',
rating: 1,
col: 'red',
dob: '19/02/1984',
car: 1
},
{
id: 2,
name: 'Mary May',
progress: 1,
gender: 'female',
rating: 2,
col: 'blue',
dob: '14/05/1982',
car: true
},
{
id: 3,
name: 'Christine Lobowski',
progress: 42,
gender: 'female',
rating: 0,
col: 'green',
dob: '22/05/1982',
car: 'true'
},
{
id: 4,
name: 'Brendon Philips',
progress: 100,
gender: 'male',
rating: 1,
col: 'orange',
dob: '01/08/1980'
},
{
id: 5,
name: 'Margret Marmajuke',
progress: 16,
gender: 'female',
rating: 5,
col: 'yellow',
dob: '31/01/1999'
},
{
id: 6,
name: 'Frank Harbours',
progress: 38,
gender: 'male',
rating: 4,
col: 'red',
dob: '12/05/1966',
car: 1
}
];
//initialize table
var table = new Tabulator.Tabulator(this.table.nativeElement, {
data: tabledata, //assign data to table
autoColumns: true //create columns from data field names
});
}
}
And yes ! that’s pretty much everything on how to import Vanilla JavaScript libraries in your Angular2+ project !
Hope it helped you or guided you through a problem ππ
Leave your comments and feedback π
Make sure to check everything in here ππ
Have a vanilla potato π₯
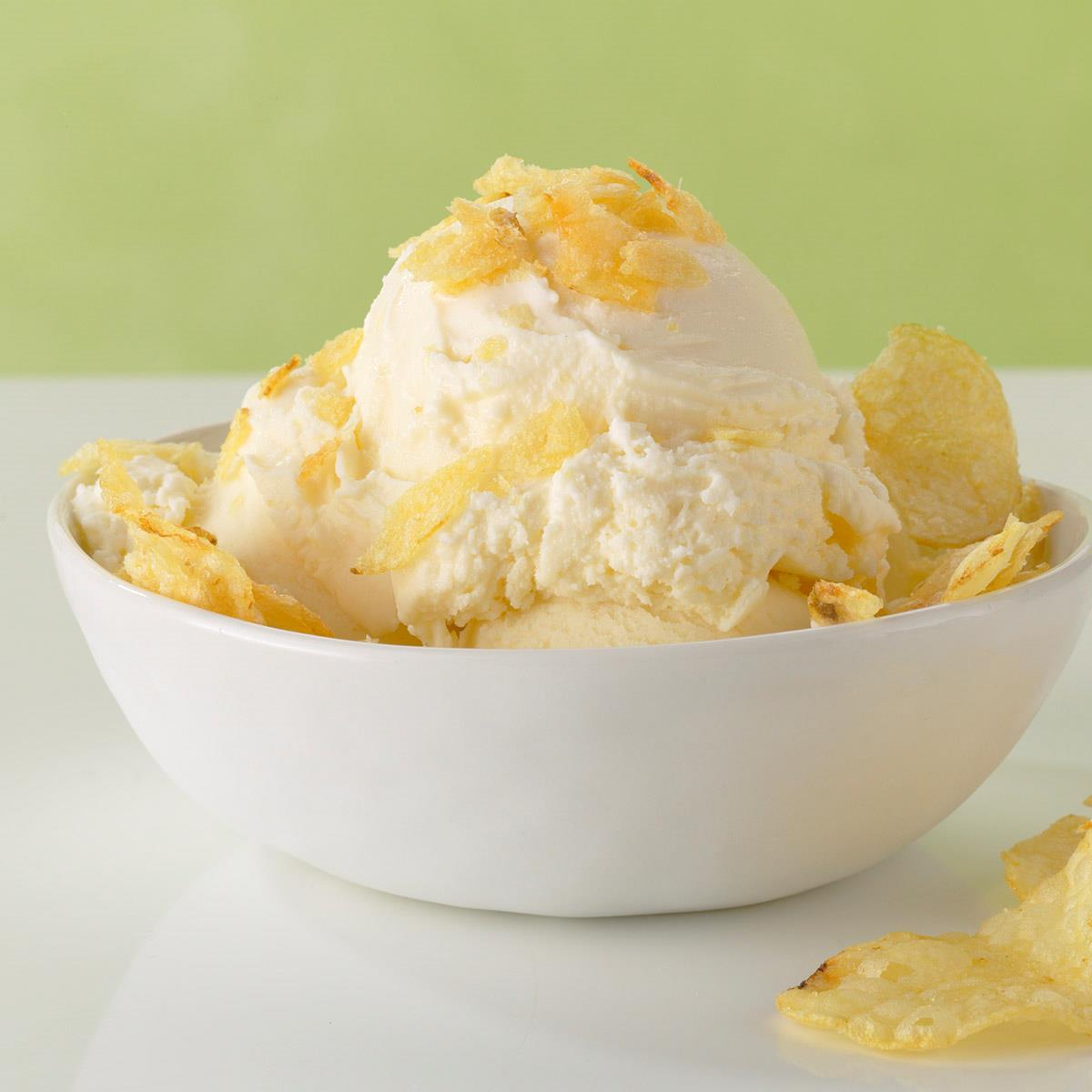
Thank you, this helped me!
Thanks Jeison, glad it did ! π