Web Components
When we think of web components, we always jump to our new modern next gen frameworks like, Angular, React, Vue … etc.
But what if we can create components, without using a framework.
Is it good?
Well, should we use a framework in the first place?
That’s a tough question, what does a framework give us?
It handles a lot of things for us:
- Clean code
- Routing
- Testing frameworks
- Versioning
- Encapsulation
But if we want to go away from it and just stick to simple vanilla HTML, CSS and JS we can definitely go to Web Components.
Myth
So, is it better than using a framework? or should I use a framework?
This is a great myth, it’s just different teams.
Angular is not better than React, and React is not better than Vue, and Vue is not better than Angular, and all of them are not better than Web Components.
Everything is good and everything is working, you just choose based on your experience, the nature of your project, and what are you most comfortable with.
Ex: you want to make a static website, will you create backend for it? NO !, don’t over engineer things.
So, just break down your project and see what’s the best approach for it based on your experience and interests. Nothing is better than anything else.
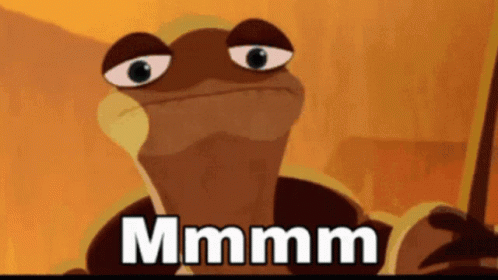
How to start
It’s super simple, we have to do two steps:
- Create a class for our component
- Define our web component
Creating the class
class HelloWorldComponent extends HTMLElement {
constructor() {
super();
}
connectedCallback() {
this.innerHTML = `<h1>Hello world!</h1>`
}
}
That’s a simple javascript class, what does this mean?
The constructor is just the constructor and we have to add super()
to it at the beginning of it.
the connectedCallback
is the first method that gets called once the element is loaded, we can see here that it replaced the innerHTML of the element with an h1 tag.
Defining the web component
customElements.define("hello-world", HelloWorldComponent)
That’s how we simply define the component. we call customElements.define and we pass the tag as well as the class we created.
LifeCycle
So, as a started, we know the first first event in the life cycle of a web component, the connectedCallback
So what is our life cycle?
Constructor
The constructor is basically a method to initialize an object instance of the class and it’s the first method to be called in initializing.
You can also assign values there.
connectedCallback
This will be called every time the component is attached to the DOM, so everytime we create a new instance of our class/component, the connectedCallback will be called.
attributeChangedCallback
This callback will be called each time an attribute is changed within the component, and it’s written like this
attributeChangedCallback(attrName, oldVal, newVal) {
// code goes here
}
disconnectedCallback
This is called once the element / component is removed from the DOM
Ex:
Some code is called to fire this line of code
document.body.removeChild(document.getElementById("hello-world"));
so, the disconnectedCallback is called, and here it is:
disconnectedCallback() {
// code goes here
}
And it’s basically used to clear resources, like unsubscribing, removing any unwanted listeners … etc
So, a complete web component should look like this:
class HelloWorldComponent extends HTMLElement {
constructor() {
super();
}
connectedCallback() {
this.innerHTML = `<h1>Hello world!</h1>`
}
attributeChangedCallback(attrName, oldVal, newVal) {
// code goes here
}
disconnectedCallback() {
// code goes here
}
}
customElements.define("hello-world", HelloWorldComponent)
Conclusion
So, that’s basically a web component, you can create and nest as many web components as you wish, and try to break down your application into small pieces as much as you can.
Think of a chat application
You can have a web component for each one of the bellow components:
- Chat box
- Message input
- Message item
- Chat settings
- Chat window
The chat box should combine all of these together and handle the communication.
And of course, try to make each web component in a separate javascript file.
Hope that was helpful, looking forward for your comments 🤠also, feel free to surf everything in here !
Here’s a potato as a Web Component.
Are these web components the base for angular component structure ?
Yes, Angular elements are Angular components packaged as custom elements
Yes Moselhy 😎