Google reCAPTCHA with Angular
You created your Angular app, of course with some forms and contact us forms, and people started to hop over your website and contacting you, some of them are just spam and running bots, you start to realize you need to install Google reCAPTCHA to avoid bots and spams.
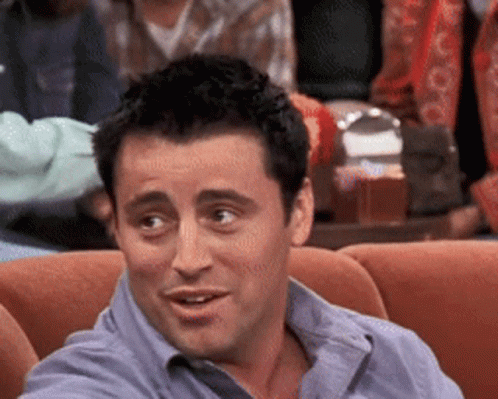
Let’s Jump Technically
Get Google reCAPTCHA Keys
Of course here we will be using the service from Google, how? we will jump to Google Console to get our keys:
https://www.google.com/recaptcha/admin
On the top right corner, click on the Plus icon to create a new site.
Let’s call it anything, for now, we call it, my-recaptcha
You can select the type of the recaptcha that you want:
- Version 3
- Version 2
- I’m not a robot
- Invisible
- Challenge
For this post, we will go through the invisible one
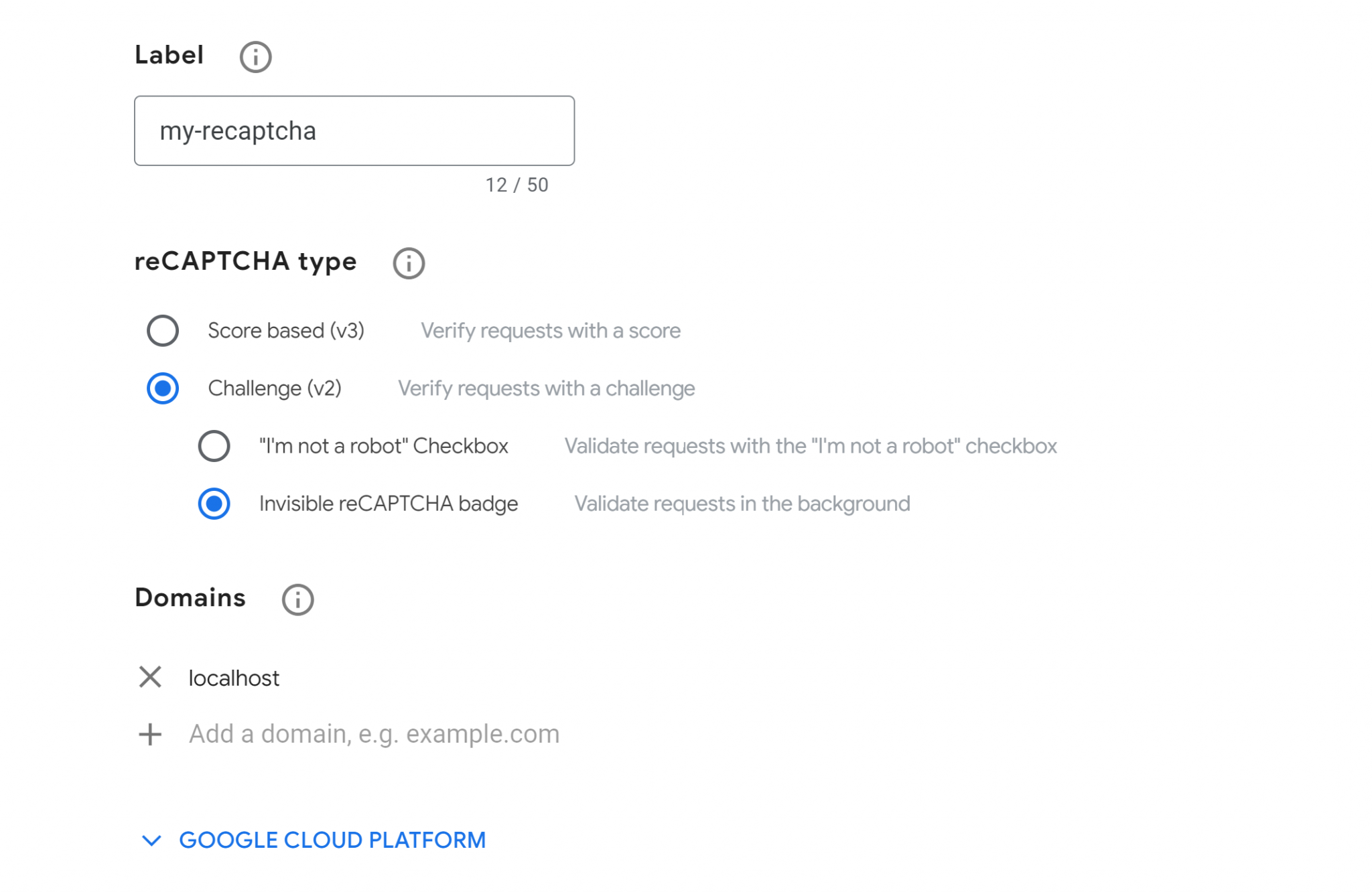
Notice: here we added localhost in the domain, so that we can test it locally, once you go live, you will need to change that to your domain, like: josepham.me
Click Submit and check the private and public keys you are provided with.
Public Key
The public key is used to be sent to your server or to google to verify the use that s/he is not a bot, or a spam.
Private Key
The private key is used on your server if you are going down the approach that you will skip google and that you decided to do the validation through your server, not client side only.
// So, for now, store the Public Key
Google reCAPTCHA with Angular
There’s a good library that we will use called ng-recaptcha
Let’s Create a New App
- Open the terminal and let’s create a new app
- Assuming you have NG Angular CLI installed globally
- $ ng new my-recaptcha
- Go through the CLI
- $ npm i ng-recaptcha
- Notice: The versions in the ng-recaptcha documentation, install the version assigned to your used Angular version
- Here, I’m using Angular16, so I should install version 12
- $ npm i ng-recaptcha@12
- Add the below HTML with your public key
- Add the RecaptchaModule in the module you are using the captcha in
- In my case, the AppModule
AppModule
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { RecaptchaModule } from 'ng-recaptcha';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,
RecaptchaModule, // <-- Here
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
app-component.html
<re-captcha (resolved)="resolved($event)" [siteKey]="publicKey"></re-captcha>
Once you save, you will find it looking like this:
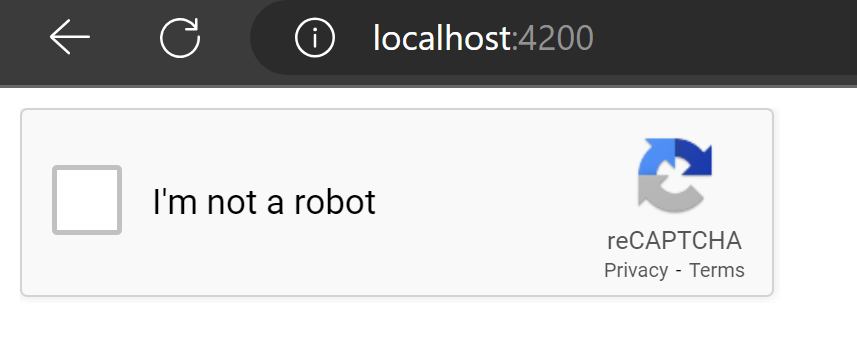
Ok, it looks cool, But I want to make it invisible, How?
Making reCAPTCHA Invisible
How? easy, just add the size=”invisible” to the html
<re-captcha size="invisible" (resolved)="resolved($event)" [siteKey]="publicKey"></re-captcha>
You will find it in the bottom right corner, but it still appears, you don’t want it right? we can play with CSS around to fix that, how?
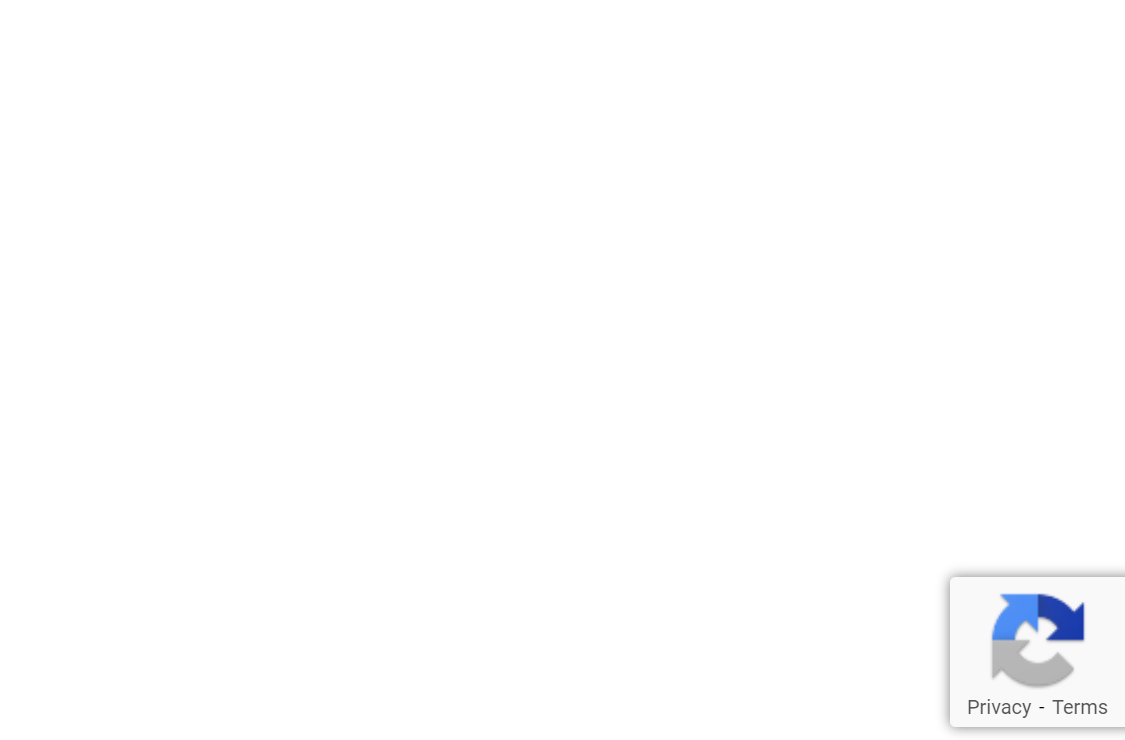
We can add those lines to our site.css
.grecaptcha-badge {
opacity: 0;
}
You will find that it disappeared !!
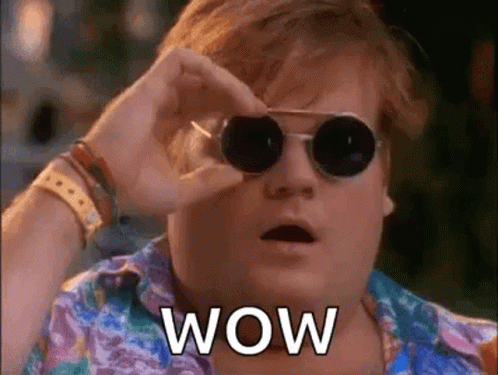
Ok, now there’s an issue, the Invisible reCAPTCHA need to be executed manually, how? we need to call an execute function. How do we do that?
We need to add a reference to the html element and then call the execute function either on
The component OnInit
OR
The click on the submit of the form
Ok, how?
We modify the HTML like so
<re-captcha #captchaRef="reCaptcha" size="invisible" (resolved)="resolved($event)" [siteKey]="publicKey"></re-captcha>
<button (click)="captchaRef.execute()">
Execute ME !
</button>
And the component.ts to be like this
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'my-recaptcha';
publicKey = 'my-very-big-public-key';
resolved(e: any) {
console.log(e);
}
}
And that’s it, you will find it logs the success that it’s resolved.
Thanks for reaching down here, looking to discuss it further with your.
Question
Do you think it should be executed on the OnInit of the component?